How to Create a Voice Call Survey System with Twilio and Go
Time to read: 5 minutes
How to Create a Voice Call Survey System with Twilio and Go
Understanding how your customers feel about your services or products is crucial for making informed decisions to improve them. It helps you identify which ones interest your customers and which do not. This is why most companies collect feedback from customers after they have used their products or services.
When collecting survey data from customers, the most commonly used method is to send them a link to fill out their responses or provide feedback. While this method is sometimes effective, it can be time-consuming for customers to type their responses. However, with the voice call survey method, customers only need to respond to survey questions verbally, making it a more time-efficient option.
In this tutorial, you’ll learn how to create an interactive voice call survey system using Twilio and Go, as well as view the statistics of the collected survey responses.
Prerequisites
To follow along with this tutorial, you'll need the following:
- Go v1.23 or later
- Access to a MySQL database, and a MySQL client such as the MySQL Command-Line Client or DataGrip for MySQL d evelopers
- A Twilio account (free or paid), with an active phone number capable of making and receiving calls
- ngrok installed on your computer and an ngrok account
- Your preferred code editor for writing Go
Create a new Go project
To get started, let's initialize a new Go project. To do that, open your terminal, navigate to the desired project directory, and run the following commands.
After running the above commands, open your preferred code editor and open the new project folder.
Create environment variables for the credentials
Let’s create environment variables to store the application’s credentials, such as Twilio credentials and the ngrok base URL. To do this, in the project's root directory, create a .env file, and add the following environment variables.
Now replace the <db_username>
and <db_password>
placeholders with your MySQL database username and password, respectively.
Retrieve your Twilio credentials
Now, let’s retrieve the Twilio credentials and set them as environment variables. Log in to your Twilio console dashboard, where you will find your Twilio credentials under the Account Info section, as shown in the screenshot below.
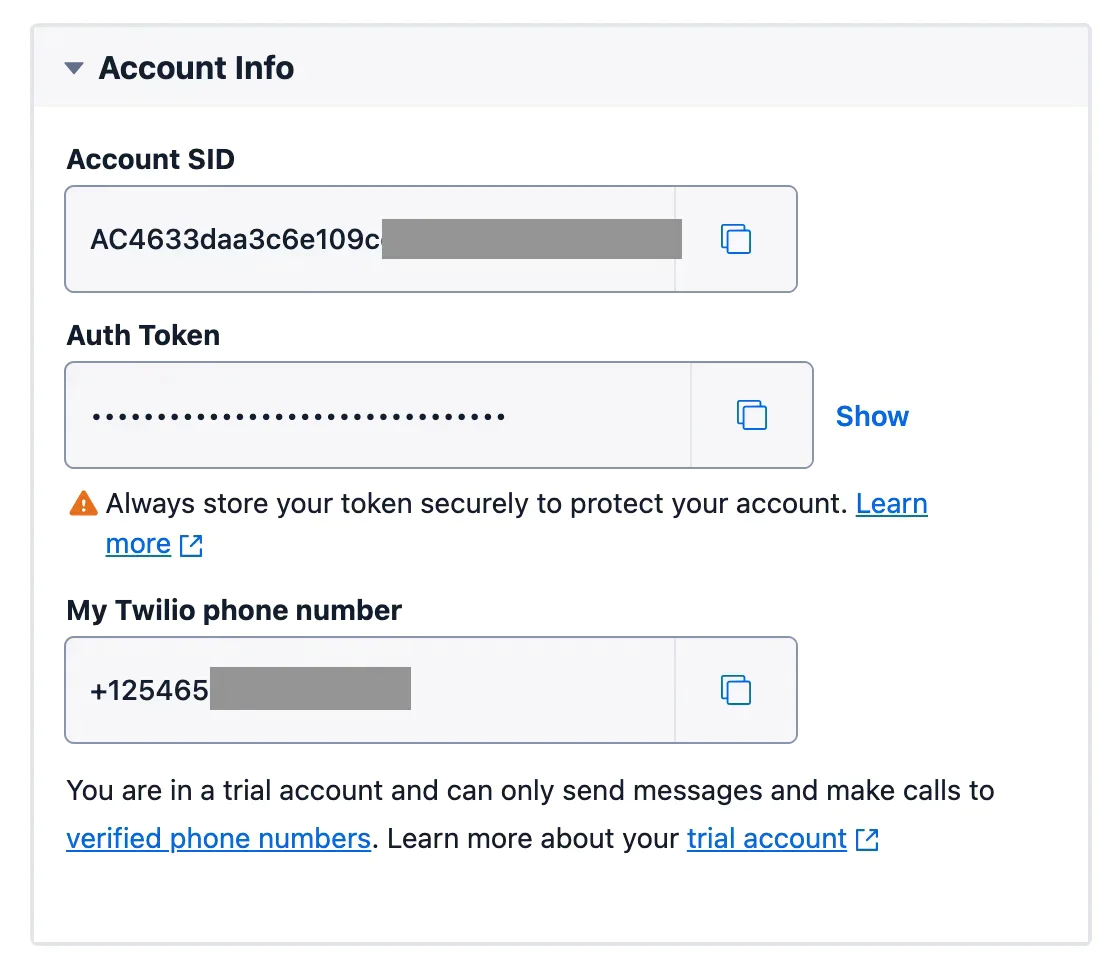
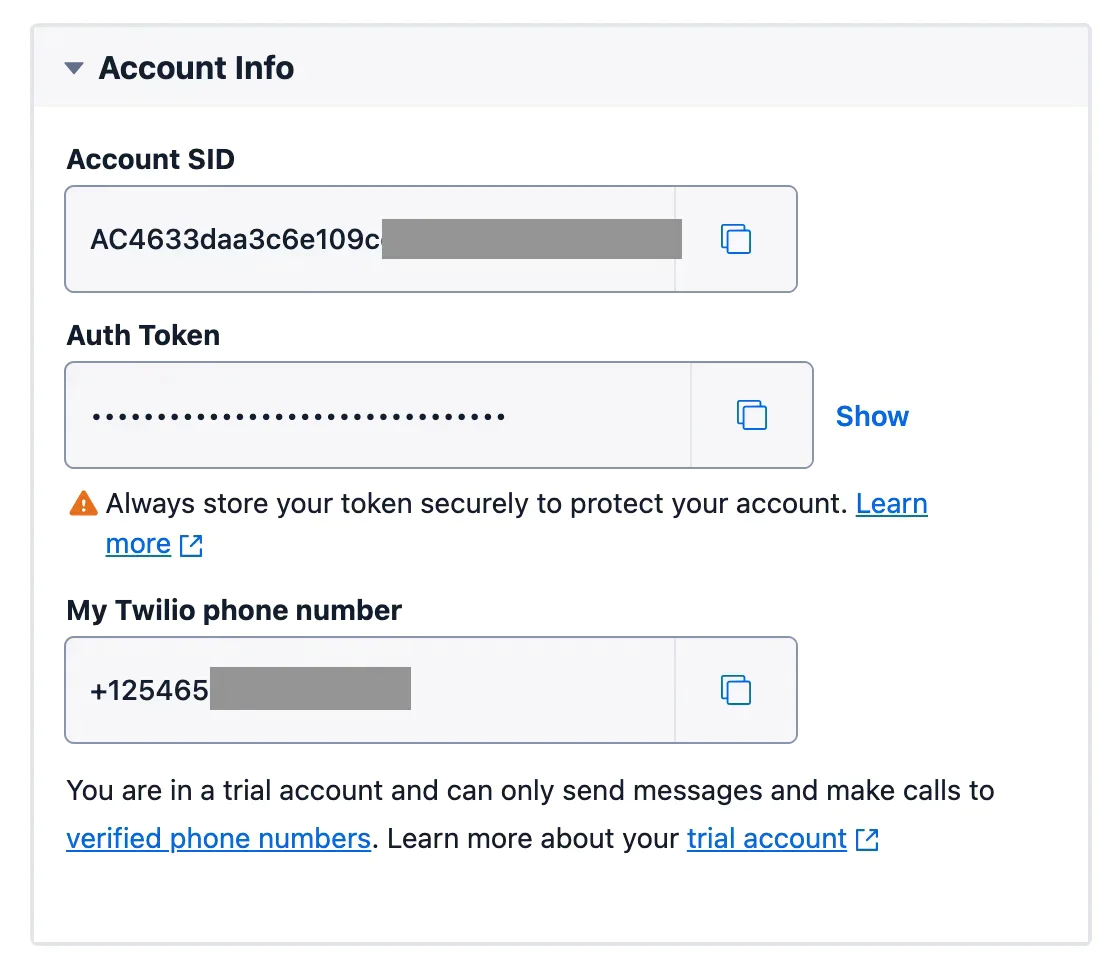
In the application’s environment variables, replace the <twilio_account_sid>
, <twilio_auth_token>
, and <twilio_phone_number>
placeholders with the corresponding Twilio Account SID, Auth Token, and phone number values.
Install Twilio's Go Helper Library
Twilio's Go Helper Library enables Go developers to seamlessly integrate Twilio services, such as sending SMS, making voice calls or other services, into applications built with the Go programming language.
To install it, run the command below in your terminal.
Install other dependencies
Let’s install the MySQL Go driver to interact with the MySQL database and the GoDotEnv library to load environment variables. To do this, run the following commands.
Define the database schema
Let's create the "customers", "survey_responses", and "survey_questions" database tables to store the survey participants' details, the survey questions, and the collected survey responses, respectively.
To do that, log in to the MySQL database server and create a new database named "voice_call_survey". Then, create the tables using the following schema definition:
The above schema consists of the following tables:
- customers: Keeps track of participants' phone numbers and survey progress
- survey_questions: Stores all survey questions
- survey_response: Links responses to questions using
questionID
and tracks respondents viarespondentID
Create the survey logic
Now, let’s create the voice survey logic, allowing the admin to add survey questions, invite customers to participate via phone call, and view statistics on the collected responses. To do this, navigate to the application's root directory, create a new file named main.go, and add the following code.
In the code above:
- All the necessary dependencies are imported.
- The
initDB()
function establishes a connection to the database server using thesql.Open()
method, which accepts two parameters:mysql
, specifying the database driver, anddb_source
, which contains the database connection string loaded from the environment variable. - The
placeCall()
function initializes a Twilio client using thetwilio.NewRestClientWithParams()
method, which accepts the Twilio Account SID and Auth Token. The <Gather> verb is used in the TwiML response to collect voice input from customers. Finally, theclient.Api.CreateCall()
method is used to place a call to the customer's phone number, asking if they would like to answer the survey questions. - The
handleResponse()
function processes the participant's consent to take the survey by capturing user responses through the Twilio Voice webhook. If the participant agrees by saying "yes", their phone number is stored in the database and the first survey question is asked. - The
handleSurvey()
function gets the customer’s response through the Twilio Voice webhook, stores the answer in the database, and then processes the next questions. - The
addQuestion()
function adds new survey questions to the database, while thesurveyStats()
function retrieves collected customer responses, enabling the admin to make informed decisions. - The
main()
function serves as the entry point of the application. It loads environment variables, initializes the database connection usinginitDB()
, and sets up the application routes before starting the HTTP server.
Create the application templates
Let’s create the user interface templates for the "add_questions", "invite", and "survey_stats" pages. From the application's root directory, create a folder named templates.
To create the "add_question" interface, navigate to the templates folder, create a file named add_question.html, and add the following code.
Next, to create the "invite page" interface, create a new file named invite.html and add the following code.
And, to create the "survey_stats page" interface, create a new file named survey_stats.html and add the following code to it.
Make the application accessible on the internet
Finally, to enable the application to process survey voice responses, it must be accessible over the internet. You can use ngrok to achieve this. To do so, run the following command in your terminal.
The command will generate a Forwarding URL in the terminal output, as shown in the screenshot below.
Next, let’s add the ForwardingURL to the environment variables. To do this, open the .env file and replace the <forwarding_url>
placeholder with the generated Forwarding URL.
Test the application
Now, let’s test the voice call survey application. To do this, start the application development server. Open another terminal tab or session and run the command below.
Next, to add survey questions, open http://localhost:8080/add-question in your browser and enter your questions, as shown in the screenshot below.
To invite customers to the voice call survey, open http://localhost:8080/invite in your browser and enter the participants' phone numbers as shown in the screenshot below.
After inviting the participants, they will receive a call to answer the survey questions. After one or more have answered the survey, you can view the survey statistics by opening http://localhost:8080/survey-stats in your browser, as shown in the screenshot below.
That’s how to create a voice call survey system with Twilio and Go
Surveys help businesses identify which services or products to invest in, enhance, or discontinue by providing valuable insights into customer preferences and feedback.
In this tutorial, you learned how to create an interactive voice call survey system and analyze the collected survey responses statistics using Twilio and Go.
Popoola Temitope is a mobile developer and a technical writer who loves writing about frontend technologies. He can be reached on LinkedIn.
Survey icons created by Freepik and Phone icons created by Kalashnyk on Flaticon.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.