Listen to Motivational Quotations using C#, ASP.NET Core, and Twilio
Time to read: 5 minutes
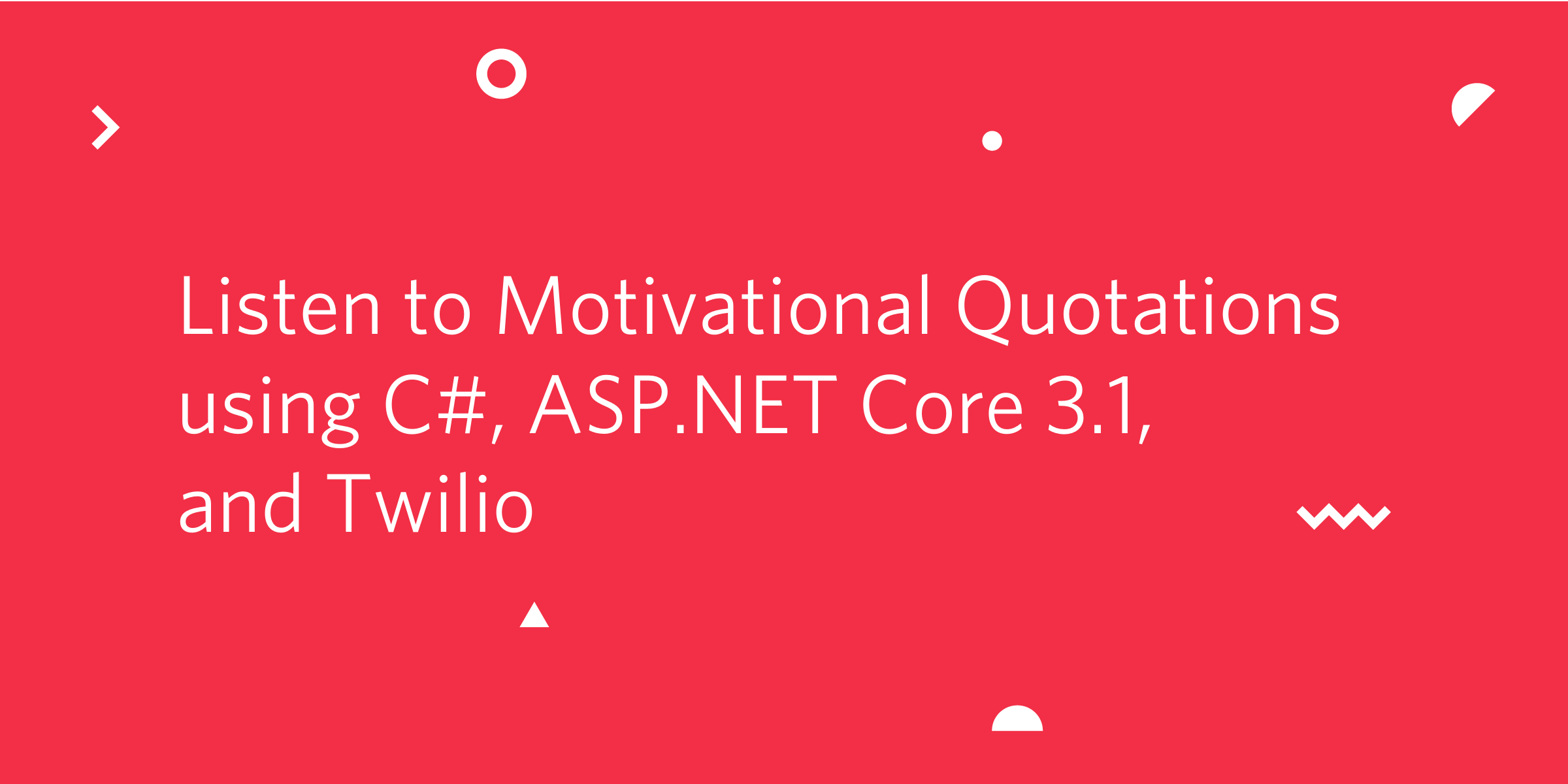
Today, customers have come to expect more personalization when interacting with businesses. Voice is one of the platforms that businesses can leverage to make the extra connection with their customers.
Twilio Programmable Voice allows you to make and receive voice calls from your applications. The Voice API includes advanced features such as creating conference calls and call monitoring.
This post will take you through the steps needed to create an ASP.NET Core application using the Twilio SDK for C# and .NET. You will build the functionality to read out a random quote when a phone call is made to a Twilio number, using the Twilio Markup Language(TwiML). Using TwiML, we will define instructions to tell Twilio what to do when an incoming call comes in.
Prerequisites
To get started with the project you will need the following:
- A Twilio account. Sign up for a free trial account and get a $10 credit.
- A call-enabled Twilio Phone Number
- The .NET Core 3.1 SDK (The SDK includes the Runtime.)
- Install ngrok to make the app visible on the internet
- Visual Studio Code, Visual Studio 2017/2019, or a code editor and the .NET CLI
To get the most out of this post, you should have knowledge of:
- ASP.NET Core
- C#
Creating the application
Create a new ASP.NET Core 3.1 Web API project and name it TwilioQuotations. If you're using the .NET CLI, the command-line instruction is as follows:
Move to the new directory created by the tooling:
Open the project in your editor/IDE. Replace the code in /Properties/launchSettings.json file with the one below:
The code above changes the port number in the applicationUrl
to a custom value to avoid clashing port numbers with other applications, and removes the sslPort
configuration to avoid issues with self-signed certificates.
Test that the server is working correctly by running it:
Visit http://localhost:3232/WeatherForecast in your browser. You should see a JSON object that begins as follows:
This indicates that the ASP.NET Core 3.1 Web API project is working properly.
Stop the application by pressing Ctrl+C in the console window where it’s running, or with the appropriate action for the editor/IDE you’re using.
Connecting to Twilio
Time to hook up the app to Twilio. Your app is running locally, but Twilio needs a publicly available endpoint. In order to access it, you will use ngrok to make the app available over the internet.
Open a new PowerShell or Windows Command Prompt console window and run the following command to start ngrok:
The output should be similar to the illustration shown below, save for the ngrok URL which is uniquely generated each time:
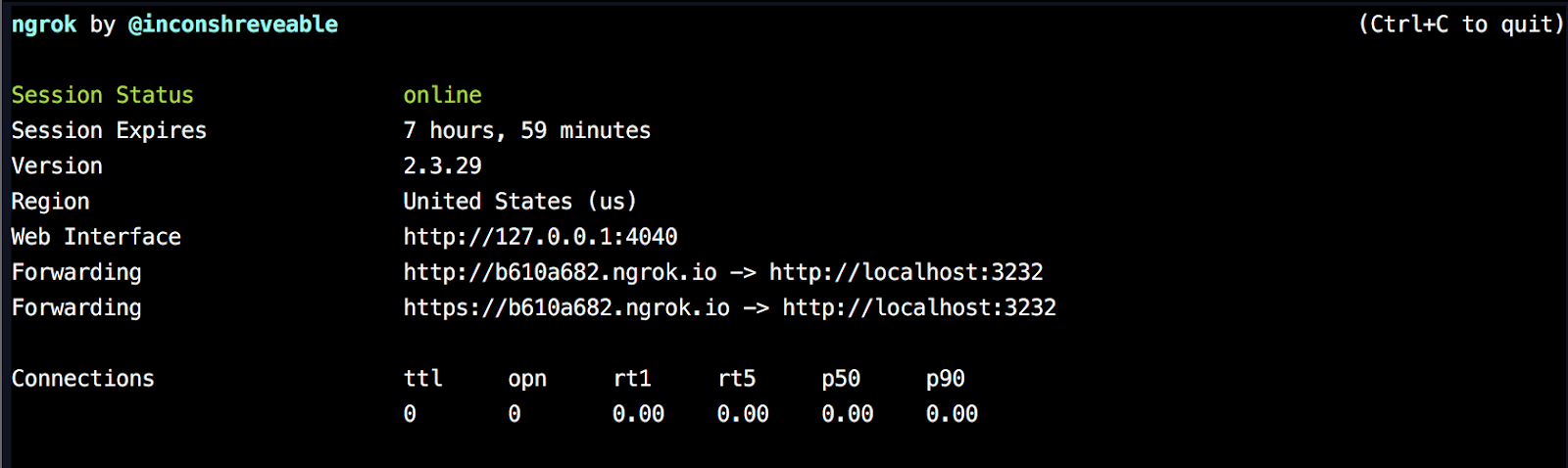
You need a call-enabled Twilio phone number to be able to receive phone calls. You can get a Twilio phone number by signing into your Twilio account and navigating to Phone Number > Buy a Number and following the instructions there.
After getting a phone number, select it in the Twilio Console under Phone Numbers > Manage Numbers > Active Numbers.
Scroll to the Voice & Fax (or Voice, depending on your location) section and add the following API endpoint to the A Call Comes In section, replacing <YOUR_NGROK_NUMBER> with the unique alphanumeric value from the Forwarding fields under Session Status in the console window in which you’re running ngrok.
Change the HTTP method for A Call Comes In from HTTP POST to HTTP GET. Your settings should be similar to those shown in the screenshot below.
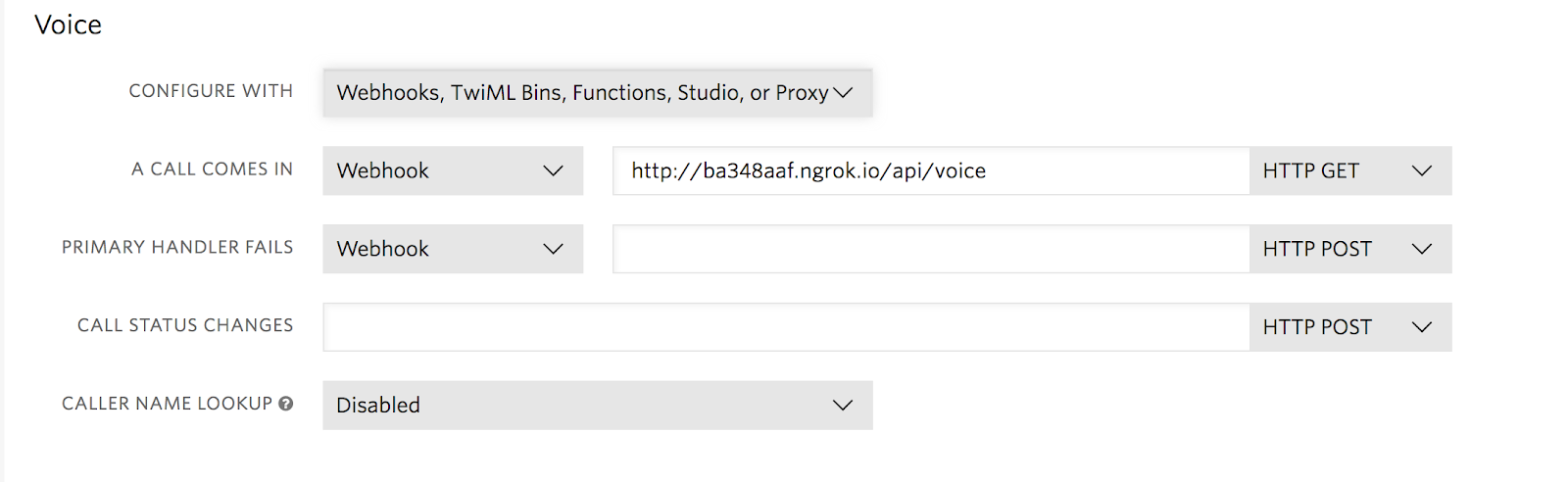
Click Save in the Twilio Console to save the change to the phone number webhook.
Leave the console window that is running ngrok open as you will use it to access the later. If you restart ngrok and generate a new url, you need to update the <YOUR_NGROK_NUMBER> portion of the URL in the Twilio console.
Now add some packages from Twilio so that we interact with the SDK.
Adding the Twilio SDK for C# and .NET
We will be using Twilio SDK for C# and the Twilio tools for ASP.NET to interact with Twilio methods. Install them with the Package Manager Console, the NuGet Package Manager, or the .NET CLI(dotnet`) by running the following commands:
Adding a web API controller
In the Controllers folder, create a new MVC Controller class file called VoiceController.cs and add the following code:
Quotations are stored in an array inside the controller. The code will randomly select a quotation from the list and will read out a message after the quotation is selected.
Start the application again with the dotnet run
command-line instruction (or the method that’s appropriate for your development environment) and go to http://localhost:3232/api/voice with your browser. You should see a quotation and the specified ending message in the browser window. You will also see the same output when you visit the Ngrok URL that you added to your Twilio Phone Number in the Twilio Console.
Trying out the app
The app is complete now and it is time to test. You can test it as long as both the localhost and ngrok servers are running.
Call your Twilio phone number. You should hear a random quotation from the list of quotations and the ending message read out!
Possible improvements
This post showed how to use the Twilio SDK in an ASP.NET Core application. There are several improvements that can be made, including:
- Connecting to a quotations api. The quotations are hard-coded in the app. You can hook it up to an api so that there are more quotes to choose from.
- Randomly selecting a voice. The
say
method accepts a parameter called voice that makes it possible to choose a voice that will read out the data. You can change it to use a different voice each time a quotation is read.
Summary
In this tutorial, you have learned how to use Twilio Programmable Voice to receive phone calls in your application. You have also learned how to use the Twilio SDK to integrate TwiML and create a voice response.
Additional resources
The following reference resources will provide you with in-depth information on some of the topics mentioned in this post:
Programmable Voice – The Twilio documentation includes quickstarts for popular programming languages, including C#, and reference information on advanced features.
TwiML™ for Programmable Voice – The documentation for TwiML, the Twilio Markup Language, will tell you all about the special XML tags you can use to build programmable voice applications.
ASP.NET Core updates in .NET Core 3.1 – Check out this blog post on the Microsoft website about the list of new features in the latest version of ASP.NET Core and learn how to upgrade an existing project.
TwilioQuest – Discover your power to change the world with this exciting and educational video game! Learn more programming and more Twilio by defeating the forces of Legacy Systems!
Brian Kabiro is a full-stack developer working with C#, Ruby, and Python. You can reach him through Twitter @briankabiro or Github briankabiro.
Related Posts
Related Resources
Twilio Docs
From APIs to SDKs to sample apps
API reference documentation, SDKs, helper libraries, quickstarts, and tutorials for your language and platform.
Resource Center
The latest ebooks, industry reports, and webinars
Learn from customer engagement experts to improve your own communication.
Ahoy
Twilio's developer community hub
Best practices, code samples, and inspiration to build communications and digital engagement experiences.